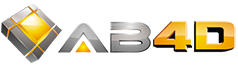 |
SceneNode Class |
SceneNode class represents a basic component of a 3D scene that is composed of various SceneNodes organized in a hierarchical structure.
Inheritance Hierarchy Namespace: Ab3d.DirectXAssembly: Ab3d.DXEngine (in Ab3d.DXEngine.dll) Version: 7.1.9105.2048 (1.0.0.0)
Syntaxpublic class SceneNode : SharedDXSceneResource
The SceneNode type exposes the following members.
Constructors | Name | Description |
---|
 | SceneNode |
Initializes a new instance of the SceneNode class.
|
 | SceneNode(String) |
Initializes a new instance of the SceneNode class.
|
Top
Properties | Name | Description |
---|
 | Bounds |
Bounds (bounding box) of this object in local coordinates (transformed by transformation on this SceneNode but not with parent's transformations).
|
 | ChildNodes |
Gets a readonly collection of child SceneNodes
|
 | ChildNodesCount |
Gets a number of child SceneNodes count
|
 | DirtyFlags |
Gets the current dirty flags
|
 | IsActuallyVisible |
Gets a Boolean that specifies if this SceneNode and its Children are actually visible taking parents visibility into account - this.IsVisible is true and also the parent is visible.
|
 | IsChildNodesListLocked |
Gets a boolean that specifies if ChildNodes for this SceneNode can be changed.
This property is set to true with calling LockChildNodes method. After locking the child nodes, they cannot be unlocked any more.
Note that this property is different from IsLocked property - that one prevent any change to SceneNode and can be unlocked.
|
 | IsHitTestVisible |
Gets or sets a Boolean that specifies if this SceneNode and all its child SceneNodes are visible to hit testing (when false, then the object cannot be hit and the testing ray passes through this SceneNode and through its child SceneNodes).
|
 | IsLocked |
Gets or sets a boolean that specified is this SceneNode can be changed or not. If true than this SceneNode cannot be changed any more.
This also prevents checking DirtyFlags and calling Update method (calling NotifySceneNodeChange has no effect).
SceneNode can be made locked with calling Lock method. It can be un-locked with calling UnLock method.
Note that we can also lock only child nodes with calling LockChildNodes method.
|
 | IsVisible |
Gets or sets a Boolean that specified is this SceneNode and its child SceneNodes are visible.
This property may be true, but if parent's SceneNode is not visible, then this SceneNode will not be actually visible (this can be get from IsActuallyVisible).
When IsVisible is set to false, the DirectX resources created with this SceneNode are not disposed (are still available).
But when rendering the RenderablePrimitive objects that are created from this SceneNode are skipped when the scene is rendered.
This makes hiding and showing ob SceneNodes very fast because only the visibility of the RenderablePrimitive is changed.
If you want to release DirectX resources when hiding the SceneNode, remove it from its parent SceneNode and call Dispose instead of setting IsVisible to false.
|
 | ParentDXScene |
Gets the parent DXScene.
|
 | ParentNode |
Gets or sets the parent SceneNode
|
 | Tag |
Tag can contain any arbitrary data.
|
 | Transform |
Gets or sets the Transformation.
|
Top
Methods | Name | Description |
---|
 | AddChild |
Adds the specified SceneNode to the ChildNodes collection of this SceneNode
|
 | AddChildren |
Adds child SceneNodes to the ChildNodes collection of this SceneNode
|
 | CleanAllDirtyFlags |
Clean dirty flags on this and child nodes.
This is called after a frame was rendered.
|
 | ClearChildNodes |
Removes all SceneNodes from the ChildNodes collection
|
 | FindNode |
Searches the ChildNodes recursively and returns the SceneNode with the specified name if found; else returns null.
|
 | ForEachChildNodeT |
ForEachChildNode calls the childNodeFoundAction for each SceneNode of type T that is find in the hierarchy of the child SceneNodes starting at this SceneNode's ChildNodes.
|
 | GetAllDescendantNodes |
Returns an enumerable of this SceneNode and all SceneNode that are children of this SceneNode and its children - gets all SceneNode in the hierarchy tree below this SceneNode as flat IEnumerable.
|
 | GetChildNode |
Returns a child SceneNode object with the specified index.
|
 | GetDetailsText |
Returns string that tells details about this SceneNode
|
  | GetSceneNodesBounds |
Returns the Bounds of the scene nodes that are children of the rootSceneNode. The sceneNodesFilter Func can be used to choose which scene nodes to use.
|
 | GetTotalTransformMatrix |
Returns a SharpDX.Matrix that represents the transformation from the Root SceneNode to this SceneNode.
The includeThisTransform parameter specifies if transformation from this SceneNode is also included in the returned matrix.
|
 | InsertChild |
Inserts the specified SceneNode to the ChildNodes collection to the specified index
|
 | Lock |
Locks the SceneNode which make it unchangable.
Note that Lock can be called only when the DirtyFlags are Unchanged - there are no changes made on this SceneNode.
|
 | LockChildNodes |
LockChildNodes method locks ChildNodes and prevents changing them. After locking SceneNodes they cannot be unlocked again.
This is used mostly by SceneNodes that does not contain any ChildNodes - for example for MeshObjectNode.
|
 | NotifyAllChildSceneNodesChange |
Add the changeType to all child SceneNodes.
This does not change this SceneNode's dirty flags.
|
 | NotifyAllParentSceneNodesChange |
Add the changeType to all parent SceneNodes.
This does not change this SceneNode's dirty flags.
|
 | NotifySceneNodeChange |
Add the SceneNodeDirtyFlags to this SceneNode's DirtyFlags flags.
This methods also calls NotifyChange on parent DXScene.
|
 | RemoveChild |
Removes the specified SceneNode from the ChildNodes collection
|
 | RemoveChildAt |
Removes the SceneNode at the specified index from the ChildNodes collection
|
 | ReplaceChild(Int32, SceneNode) |
Replaces the SceneNode at the specified index with the newChildNode
|
 | ReplaceChild(SceneNode, SceneNode) |
Replaces the currentChildNode with the newChildNode
|
 | ToString |
Returns a String that represents this instance.
(Overrides ObjectToString) |
 | UnLock |
Unlocks the SceneNode.
|
 | Update |
Update method is called on each update phase (before render phase)
|
 | UpdateBounds |
Updates the bounds of this SceneNode if the dirty flags indicates that the bounds could be changed of if the forceUpdate parameter is set to true
|
 | UpdateWorldBoundingBox |
Updates the worldBounds field based on the current worldMatrix and Bounds.
|
 | UpdateWorldMatrix |
UpdateWorldMatrix checks if dirty flags indicate that the final world matrix for this SceneNode needs to be recalculated.
|
Top
Events | Name | Description |
---|
 | ChildNodesChanged |
Occurs when list of ChildNode is changed.
|
 | ParentNodeChanged |
Occurs when ParentNode is changed.
Occurs also when this SceneNode is added to the SceneNodes tree for the first time or when it is removed from the SceneNodes tree (ParentNode is set to null).
This event does not occur when this SceneNode is disposed.
|
 | ResourcesInitialized |
ResourcesInitialized event is fried after the OnInitializeResources was called and the DirectX resources were created.
|
Top
Fields | Name | Description |
---|
 | IsWorldMatrixIdentity |
True if final world matrix (WorldMatrix) is identity.
This field can be checked to skip multipliyng with WorldMatrix.
|
 | WorldBounds |
Bounds with BoundingBox in world coordinates (transformed by parents transformations and transformation on this SceneNode).
|
 | WorldMatrix |
When IsWorldMatrixIdentity is false this field defines the final world matrix that is calculated from the parent's world matrix and this node's transformation matrix.
When IsWorldMatrixIdentity is true this field has an invalid value (is not set to Identity matrix for performance reasons).
|
Top
Extension Methods | Name | Description |
---|
 | GetGeometryModel3D |
Returns a WPF's GeometryModel3D that was used to create the specified sceneNode.
If the sceneNode does not define a GeometryModel3D object, then null is returned.
(Defined by Extensions) |
 | GetModel3D |
Returns a WPF's Model3D that was used to create the specified sceneNode.
If the sceneNode does not define a Model3D object, then null is returned.
(Defined by Extensions) |
 | GetModelVisual3D |
Returns a WPF's ModelVisual3D that was used to create the specified sceneNode or any parent SceneNode (in case searchParentSceneNodes is true).
If a SceneNode that defines a ModelVisual3D is not found, then null is returned.
(Defined by Extensions) |
 | GetVisual3D |
Returns a WPF's Visual3D that was used to create the specified sceneNode or any parent SceneNode (in case searchParentSceneNodes is true).
If a SceneNode that defines a Visual3D is not found, then null is returned.
(Defined by Extensions) |
Top
See Also